Showing
- models/res_partner.py 23 additions, 23 deletionsmodels/res_partner.py
- models/ur_financial_system.py 12 additions, 10 deletionsmodels/ur_financial_system.py
- models/ur_month_timesheet.py 102 additions, 0 deletionsmodels/ur_month_timesheet.py
- models/ur_regional_convention.py 12 additions, 10 deletionsmodels/ur_regional_convention.py
- report/report_hr_timesheet.xml 61 additions, 39 deletionsreport/report_hr_timesheet.xml
- report/report_hr_timesheet_act.xml 138 additions, 0 deletionsreport/report_hr_timesheet_act.xml
- security/ir.model.access.csv 4 additions, 0 deletionssecurity/ir.model.access.csv
- security/security_rules.xml 54 additions, 57 deletionssecurity/security_rules.xml
- static/description/icon.png 0 additions, 0 deletionsstatic/description/icon.png
- static/src/css/style.css 4 additions, 4 deletionsstatic/src/css/style.css
- static/src/js/ur_month_timesheet.js 31 additions, 0 deletionsstatic/src/js/ur_month_timesheet.js
- static/src/xml/month_timesheet.xml 36 additions, 0 deletionsstatic/src/xml/month_timesheet.xml
- views/assets.xml 18 additions, 7 deletionsviews/assets.xml
- views/cgscop_timesheet_code.xml 8 additions, 6 deletionsviews/cgscop_timesheet_code.xml
- views/cgscop_timesheet_sheet.xml 168 additions, 58 deletionsviews/cgscop_timesheet_sheet.xml
- views/hr_timesheet.xml 352 additions, 75 deletionsviews/hr_timesheet.xml
- views/hr_timesheet_cgscop.xml 135 additions, 48 deletionsviews/hr_timesheet_cgscop.xml
- views/res_company.xml 30 additions, 0 deletionsviews/res_company.xml
- views/res_partner.xml 30 additions, 25 deletionsviews/res_partner.xml
- views/ur_financial_system.xml 15 additions, 7 deletionsviews/ur_financial_system.xml
models/ur_month_timesheet.py
0 → 100644
report/report_hr_timesheet_act.xml
0 → 100644
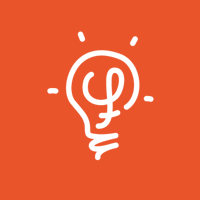
| W: | H:
| W: | H:
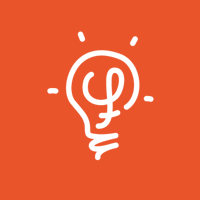
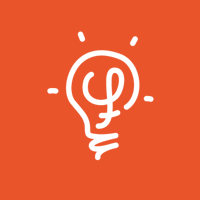
static/src/js/ur_month_timesheet.js
0 → 100644
static/src/xml/month_timesheet.xml
0 → 100644
This diff is collapsed.
views/res_company.xml
0 → 100644